C Programming Best Practices Pdf
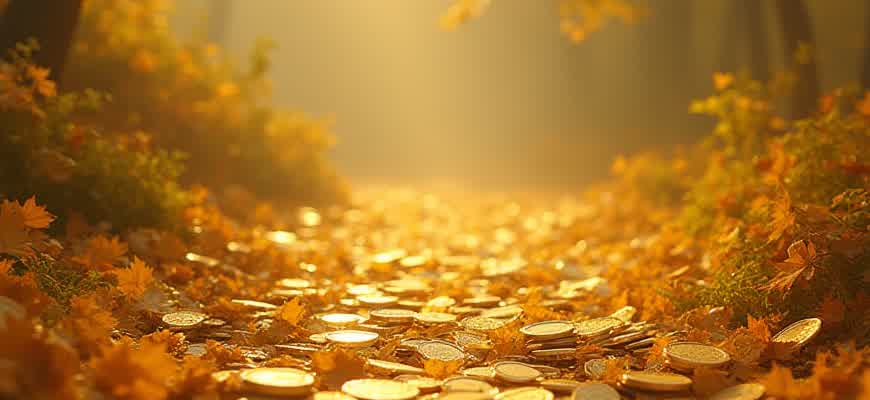
Effective programming in C requires adherence to certain principles that enhance code readability, maintainability, and performance. Below are key practices that are widely regarded as fundamental for writing clean and efficient C code.
Key principles for writing good C code:
- Proper code organization: Keep functions short, focused, and cohesive.
- Consistent naming conventions: Use meaningful and descriptive names for variables, functions, and constants.
- Minimize global variables: Limit their scope to prevent potential issues with data integrity.
Common mistakes to avoid:
- Ignoring compiler warnings: These often point to potential bugs or bad practices.
- Excessive use of pointers without proper checks: This can lead to memory leaks or segmentation faults.
- Not using proper error handling: Ensure that every function that can fail is adequately protected.
Tip: Always prefer
const
when declaring variables or parameters that should not be modified, as it improves code clarity and reduces the risk of accidental changes.
Table of essential C coding concepts:
Concept | Description |
---|---|
Memory Management | Ensure proper allocation and deallocation of memory to prevent memory leaks. |
Pointer Arithmetic | Handle pointers with caution and always check for null references before dereferencing. |
Modular Design | Divide the code into manageable modules to enhance reusability and maintainability. |
C Programming Best Practices Guide
When programming in C, adhering to best practices is essential for writing clean, efficient, and maintainable code. Following these practices helps reduce errors, ensures better performance, and improves the readability of the code. As C is a low-level language, careful attention to details like memory management, data types, and modular design is crucial. This guide will focus on key practices that can make a significant difference in your C programming projects.
By integrating these strategies into your coding routine, you will not only enhance the quality of your work but also ease the debugging and testing processes. The following best practices, outlined in this document, are based on common industry standards and community recommendations. These guidelines cover areas such as code organization, efficient memory usage, error handling, and more.
Key Best Practices
- Use Meaningful Names – Always choose descriptive names for variables, functions, and constants. This improves code readability and makes maintenance easier.
- Avoid Global Variables – Global variables can introduce hard-to-find bugs and reduce the modularity of your code. Use local variables and pass data through function parameters.
- Comment Wisely – Comments should explain why certain logic is used, not what it does. This provides valuable context for future developers.
Memory Management
- Initialize Variables – Always initialize variables before use to prevent unpredictable behavior.
- Free Allocated Memory – After using dynamic memory allocation (e.g., using malloc), ensure to free up memory to avoid memory leaks.
- Check for Null Pointers – Always verify that pointers are not NULL before dereferencing them.
Structure and Modularity
Designing your C program with structure and modularity is vital. Keep your functions small and focused on a single task, and use header files to separate declarations from implementations. This helps in testing individual components without affecting the whole codebase.
Tip: Avoid large, monolithic functions. Break down tasks into smaller, reusable functions to maintain clarity and reusability.
Example of Proper Function Use
Function | Purpose |
---|---|
int add(int a, int b) | Adds two integers and returns the result. |
void print_result(int result) | Prints the result to the console. |
Improving Code Readability with Clear Naming Conventions
In C programming, naming conventions play a significant role in ensuring code is understandable and maintainable. When functions, variables, and constants are named logically and descriptively, it becomes easier for developers to quickly grasp the purpose of the code. This reduces the cognitive load required to work with the codebase, promoting quicker debugging and better collaboration among team members.
By following consistent naming conventions, developers can avoid ambiguity and prevent potential confusion. Clear names provide valuable context, making the code self-explanatory, even to those unfamiliar with the project. This practice also aids in reducing the time spent on documentation, as meaningful names reduce the need for excessive comments.
Key Principles for Naming Conventions
- Descriptive and Specific: Names should convey the exact purpose or function. For example, use calculateTotalAmount() instead of calc() or foo().
- Consistency: Maintain uniformity in naming across the project. For example, if camelCase is used for function names, avoid mixing it with snake_case.
- Avoid Abbreviations: While abbreviations might seem quicker, they can confuse other developers. For instance, use numberOfItems instead of numItems.
Examples of Effective Naming
Bad Naming | Good Naming |
---|---|
int a; | int itemCount; |
void f(); | void calculateTotal(); |
Important: Meaningful names are especially important for long-term projects, where future modifications are frequent. A well-named function or variable can save time during code review and debugging sessions.
Memory Efficiency Techniques in C Programming
Efficient memory management is critical in C programming, especially when working with large datasets or on resource-constrained systems. Understanding how to allocate, access, and free memory can significantly improve the performance of your programs. Mismanagement of memory, such as memory leaks or inefficient use of memory resources, can lead to slow performance, crashes, or system instability.
To optimize memory usage in C, it’s essential to use appropriate data structures, minimize the usage of dynamic memory allocation, and free memory once it is no longer needed. The following strategies can help programmers effectively manage memory consumption in C.
Key Techniques for Memory Optimization
- Use of Static Memory Allocation: Where possible, prefer static memory allocation over dynamic memory. This avoids the overhead of using functions like malloc or free.
- Memory Pooling: Pre-allocate a fixed amount of memory and manage it manually to avoid frequent allocation and deallocation, which can be costly in terms of performance.
- Minimize Fragmentation: Keep track of memory fragmentation, especially when allocating and freeing blocks of memory. Consider using memory allocators designed to reduce fragmentation.
Best Practices for Dynamic Memory
- Always Check Allocation Success: Ensure that memory allocation functions (malloc, calloc) are checked for successful allocation before using the memory.
- Free Allocated Memory: Always use free() to release dynamically allocated memory when it is no longer needed to avoid memory leaks.
- Use Smart Pointers or Wrappers: In more complex applications, consider using smart pointers or wrappers around malloc/calloc/free to automate memory management and avoid common mistakes.
Proper management of memory in C can significantly enhance performance and avoid costly runtime errors. Always be conscious of how memory is allocated and freed within your programs.
Memory Usage Summary
Strategy | Benefit | Considerations |
---|---|---|
Static Allocation | Faster, no runtime allocation | Limited flexibility |
Memory Pooling | Reduces allocation overhead | Requires careful management |
Dynamic Allocation | Flexible, adaptable to needs | Must ensure proper deallocation |
How to Avoid Common Pitfalls in C Programming
C programming is powerful but prone to common errors that can lead to undefined behavior, crashes, or memory leaks. Avoiding these mistakes requires a solid understanding of C’s features and nuances. The following practices will help you write safer, more efficient C code and reduce the chance of errors.
By understanding some of the most frequent mistakes, you can prevent issues related to memory management, pointer arithmetic, and the use of uninitialized variables. Here are some strategies to keep in mind when coding in C.
Key Strategies for Error Prevention
- Always Initialize Variables: Uninitialized variables can lead to unpredictable results, especially when dealing with pointers. Always initialize variables before use.
- Check for Null Pointers: Dereferencing a null pointer can cause crashes. Always ensure that pointers are not null before dereferencing them.
- Use Proper Memory Allocation: Be cautious with dynamic memory allocation. Always check the result of
malloc
orcalloc
for a successful allocation before using the allocated memory.
Common Mistakes and How to Fix Them
- Forgetting to Free Memory: Memory leaks occur when dynamically allocated memory is not freed. Always pair
malloc
withfree
to ensure proper memory management. - Incorrect Pointer Arithmetic: Pointer arithmetic can be tricky. Ensure you understand how the pointer increments work based on the type of data it points to.
- Buffer Overflows: Avoid buffer overflows by always ensuring that buffers are large enough to hold the data they are meant to store.
Important: Always use functions like
strncpy
instead ofstrcpy
to avoid buffer overflows when handling strings.
Common Mistakes in Code
Mistake | Consequence | Solution |
---|---|---|
Uninitialized Variables | Unpredictable behavior | Initialize all variables |
Dereferencing Null Pointers | Crashes | Check for null before dereferencing |
Memory Leaks | Memory exhaustion | Always free allocated memory |
Best Practices for Error Handling in C Code
Effective error management is crucial in C programming to ensure the stability and reliability of the application. C does not offer built-in exception handling mechanisms like other languages, so developers must rely on strategies such as return codes, global variables, and external libraries to capture and handle errors.
Implementing proper error handling techniques can greatly improve the maintainability and debugging process of the code. By using consistent error-checking patterns and informative logging, developers can quickly identify and resolve issues during development and runtime.
Common Approaches for Error Handling
- Return codes: Functions return specific error codes that the caller checks to determine whether an operation was successful or failed. This is one of the most common methods in C.
- Global variables: Using global error flags or variables to track the status of various operations. This can be helpful for centralized error management but may introduce concurrency issues.
- External libraries: Using third-party libraries like
errno
orsetjmp/longjmp
for handling errors in a more structured way.
Best Practices
- Always check function return values: C functions typically return values to indicate success or failure. For example, check whether memory allocation succeeds by verifying if the return value of
malloc
isNULL
. - Use descriptive error messages: When an error occurs, print or log meaningful messages that describe the cause and location of the failure. This makes debugging faster and more efficient.
- Handle errors early: Fail fast by detecting and handling errors at the point where they occur, rather than allowing them to propagate unchecked.
- Use
errno
for system-level errors: For system calls and library functions that useerrno
, always check its value after an operation that could fail. - Avoid silent failures: Never ignore errors. Even if you cannot fix the issue immediately, at least log it or return a meaningful error code.
Note: In complex systems, it's often better to define a structured error-handling strategy that includes error codes, logging, and recovery procedures. This approach ensures consistency and avoids ambiguity.
Error Handling Summary
Technique | Advantages | Disadvantages |
---|---|---|
Return codes | Simpler, widely used | Requires constant checking, no stack trace |
Global variables | Centralized error management | Potential concurrency issues, can be error-prone |
External libraries | Structured, robust handling | Dependency on third-party libraries |
Effective Approaches for Writing Modular and Reusable C Functions
When developing in C, writing modular functions that can be reused across different projects is a key strategy for efficient and maintainable code. Modular functions improve readability, reduce redundancy, and simplify debugging. To achieve this, it’s important to follow specific techniques and guidelines that promote the separation of concerns and ease of integration. By focusing on creating small, independent units of work, the overall complexity of the program can be minimized.
Additionally, reusable code saves time by eliminating the need to rewrite common logic for each new project. The main goal is to design functions that are both general and flexible, allowing them to be easily adapted to various situations. Here are some practical strategies for writing reusable and modular C functions:
Best Practices for Writing Modular C Functions
- Use Descriptive Function Names: A function's name should clearly describe its purpose. Avoid ambiguous names and ensure it represents the specific task the function performs.
- Keep Functions Small: Break down large tasks into smaller, manageable functions. Each function should ideally perform only one task, following the principle of Single Responsibility.
- Limit Global Variables: Global variables can make your code less reusable and harder to debug. Pass necessary data through function parameters instead of relying on global state.
- Define Clear Interfaces: Ensure that each function has a well-defined interface with clearly documented input and output parameters. This improves readability and reduces dependencies.
Strategies for Enhancing Reusability
- Use Parameters Effectively: Functions should accept parameters rather than hardcoding values. This allows the function to be used in various contexts.
- Return Values Instead of Printing: Avoid using functions that directly print output. Instead, return values from functions and allow the calling code to handle output.
- Modularize Error Handling: Implement error handling in a consistent way across functions, such as returning error codes or using exception mechanisms where appropriate.
Keep your functions simple, and they will serve you well across many projects.
Common Design Patterns
Pattern | Description |
---|---|
Function as a Service | Design functions that accept input data, process it, and return a result without side effects. |
Callback Functions | Allow the caller to specify certain behaviors through function pointers, increasing the flexibility of your code. |
Helper Functions | Create small, reusable utility functions that are common across different parts of your program. |
Effective Use of Pointers in C Programming
Pointers are one of the most powerful features in C programming, offering flexibility and efficiency when working with memory. Proper use of pointers can significantly improve the performance and functionality of your programs. However, pointers come with a learning curve and can lead to errors if not handled correctly. Understanding the fundamentals of pointers and their applications can prevent common pitfalls such as memory leaks or segmentation faults.
By mastering pointers, you gain direct control over memory, which allows for dynamic memory management and manipulation of data structures like linked lists and trees. Proper pointer management is essential for writing optimized, maintainable code that operates efficiently in various scenarios, particularly in low-level system programming and embedded systems.
Best Practices for Using Pointers
- Always initialize pointers: Uninitialized pointers can lead to undefined behavior. Set them to NULL or a valid memory address when declaring.
- Use pointer arithmetic cautiously: Pointer arithmetic can lead to errors if the pointer goes out of bounds or points to an incorrect memory location.
- Free allocated memory: Always use free() to deallocate dynamically allocated memory to prevent memory leaks.
- Pass by reference: Use pointers to pass large data structures (e.g., arrays) to functions to avoid unnecessary copying of data.
Common Pointer Mistakes to Avoid
- Dereferencing NULL pointers: Always check if a pointer is NULL before dereferencing it.
- Memory leaks: Ensure that every malloc or calloc call is paired with free to avoid memory wastage.
- Pointer type mismatches: Ensure the pointer type matches the data type it points to, as incorrect type casting can cause unexpected results.
Example: Pointer Usage in a Simple Program
Code Example | Description |
---|---|
int *ptr = NULL; |
Initialize the pointer to NULL to avoid undefined behavior. |
ptr = malloc(sizeof(int)); |
Allocate memory dynamically for an integer. |
*ptr = 5; |
Assign a value to the memory location pointed to by ptr. |
free(ptr); |
Deallocate the memory to avoid a memory leak. |
When using pointers in C, always remember that careful management of memory and pointer arithmetic can significantly improve the efficiency and safety of your code.
Using Compiler Warnings for Code Improvement
Compiler warnings serve as an invaluable tool for developers aiming to enhance the overall quality of their code. Rather than being ignored or dismissed, these warnings should be treated as critical signals that indicate potential issues, inefficiencies, or risky behaviors in the code. By addressing these warnings promptly, developers can prevent subtle bugs from surfacing in production environments. This proactive approach helps ensure that the code remains clean, efficient, and less prone to errors.
Incorporating compiler warnings into your development workflow allows you to identify potential problems early, reducing debugging time and enhancing maintainability. By systematically configuring your compiler to treat warnings as errors, you can enforce a stricter coding standard across the project. This discipline leads to more robust and reliable software while encouraging developers to follow best practices.
Common Types of Warnings to Address
- Unused Variables: These warnings highlight variables that are declared but not used in the code, often indicating redundant or unnecessary logic.
- Uninitialized Variables: Warnings related to uninitialized variables can prevent unpredictable behavior and logic flaws.
- Implicit Type Conversions: Compiler warnings about type conversions can help prevent precision loss or unexpected behavior due to automatic type casting.
Steps to Effectively Use Warnings
- Configure the Compiler Properly: Ensure that your compiler is set to display all warnings, including the most verbose ones.
- Treat Warnings as Errors: Use compiler flags to elevate warnings to errors, forcing developers to resolve them before code compilation succeeds.
- Review Warnings Periodically: Regularly go through warning messages, as some issues might arise in different stages of development or as the codebase evolves.
Note: Consistently addressing warnings leads to better code maintainability and fewer runtime issues.
Table of Key Compiler Warnings and Their Impact
Warning Type | Potential Issue | Impact on Code |
---|---|---|
Unused Variables | Indicates unnecessary code | Increases code complexity and size |
Uninitialized Variables | Can lead to undefined behavior | Potential for bugs or incorrect results |
Implicit Type Conversions | Automatic casting may cause data loss | Precision errors and unexpected behavior |
How to Write Secure C Code: Preventing Vulnerabilities
Writing secure C code is essential to ensure the integrity of applications and prevent potential vulnerabilities. By understanding common pitfalls and implementing best practices, developers can significantly reduce the chances of introducing security flaws in their code. Following a structured approach, focusing on proper validation, memory handling, and careful attention to detail will result in safer and more reliable programs.
Security vulnerabilities in C code can lead to critical issues such as buffer overflows, null pointer dereferencing, and improper memory management. To address these, developers must adopt a proactive mindset and adhere to secure coding principles that mitigate risks and improve the overall resilience of their software.
Key Practices to Ensure Secure C Code
- Validate Input Data: Always check and sanitize input from users or external sources to avoid injection attacks, like SQL or command injections.
- Avoid Buffer Overflows: Be cautious with string and array operations, such as strcpy, which can cause buffer overflows if not properly bounded.
- Use Memory Management Carefully: Always initialize memory before use and free it once it's no longer needed. Unused or uninitialized memory may cause undefined behavior.
- Handle Pointers Safely: Never dereference null or invalid pointers. Always check for null pointers before using them in operations.
Common Security Vulnerabilities in C
- Buffer Overflow: Occurs when more data is written to a buffer than it can hold, leading to overwriting adjacent memory.
- Use of Dangerous Functions: Functions like gets() or strcpy() do not perform bounds checking, leading to potential buffer overflows.
- Race Conditions: A situation where the program’s behavior depends on the relative timing of events, leading to inconsistent behavior or vulnerabilities.
Important Tips for Secure C Coding
Always prefer using safer alternatives such as strncpy, snprintf, and fgets instead of potentially dangerous functions like strcpy, gets, and scanf.
Additionally, consider using tools like static code analyzers to catch potential issues early in the development process. These tools can help identify common vulnerabilities, such as buffer overflows, memory leaks, and uninitialized variables.
Memory Management and Pointers
Unsafe Practice | Secure Practice |
---|---|
Dereferencing null pointers | Always check pointers before dereferencing |
Using uninitialized memory | Initialize memory before use |
Memory leaks from forgotten free() calls | Ensure proper deallocation of memory after use |